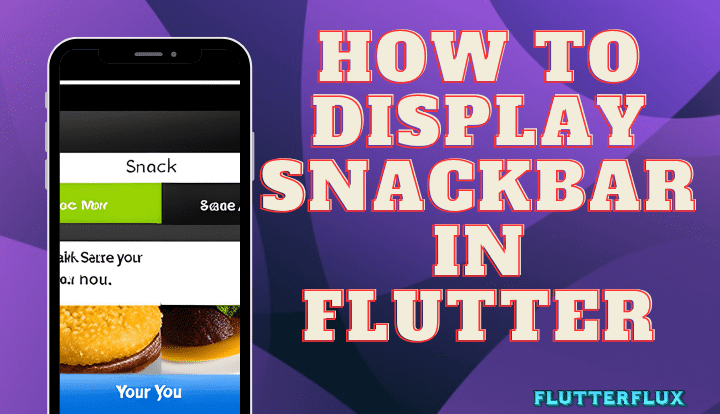
Snackbar displays small messages at the bottom of the screen. It provides user feedback after a task or error.
Flutter Material Design’s Snackbar is simple. Flutter ScaffoldMessenger
widget displays Snackbar messages. An example:
import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'flutterflux', home: HomePage(), ); } } class HomePage extends StatefulWidget { @override _HomePageState createState() => _HomePageState(); } class _HomePageState extends State<HomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Row(children: [ Image.asset( 'assets/logo.png', height: 30, ), Text('flutterflux.com') ]), ), body: Center( child: ElevatedButton( onPressed: () { ScaffoldMessenger.of(context).showSnackBar(SnackBar( content: Text('This is a floating SnackBar'), )); }, child: Text('show snackbar')), )); } }
Output

The ScaffoldMessenger
widget showSnackBar
function takes a SnackBar in Flutter widget. The SnackBar
widget displays the message, and the duration attribute controls its screen time.
Design SnackBar in Flutter
import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'flutterflux', home: HomePage(), ); } } class HomePage extends StatefulWidget { @override _HomePageState createState() => _HomePageState(); } class _HomePageState extends State<HomePage> with TickerProviderStateMixin { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Row(children: [ Image.asset( 'assets/logo.png', height: 30, ), Text('flutterflux.com') ]), ), body: Center( child: ElevatedButton( onPressed: () { ScaffoldMessenger.of(context).showSnackBar(SnackBar( duration: Duration(seconds: 2), backgroundColor: Colors.deepPurple,//snackbar backgroundcolor behavior: SnackBarBehavior.floating, action: SnackBarAction(label: 'undo', onPressed: () {}), shape: RoundedRectangleBorder( borderRadius: BorderRadius.circular(10.0), ), content: Text('This is a SnackBar'), )); }, child: Text('show snackbar')), )); } }
Output

Custom SnackBar in Flutter
Flutter SnackBar are brief popup messages that show at the bottom of the screen to notify the user of an action or event. Flutter SnackBar creation:
- Make a new
StatefulWidget
to store the SnackBar current state.
class CustomSnackBar extends StatefulWidget { final String message; CustomSnackBar({required this.message}); @override _CustomSnackBarState createState() => _CustomSnackBarState(); } class _CustomSnackBarState extends State<CustomSnackBar> { @override Widget build(BuildContext context) { return Container( height: 50, decoration: BoxDecoration( color: Colors.deepPurple, borderRadius: BorderRadius.circular(10), ), child: Row( mainAxisAlignment: MainAxisAlignment.spaceAround, children: [ SizedBox(width: 10), Text( widget.message, style: TextStyle(color: Colors.white, fontSize: 16), ), Icon(Icons.check, color: Colors.white), ], ), ); } }
Here, the ScaffoldMessenger
is used to display the SnackBar. The SnackBar in Flutter accepts a previously-created custom widget for its content parameter
Full code Custom SnackBar Flutter
import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'flutterflux', home: HomePage(), ); } } class HomePage extends StatefulWidget { @override _HomePageState createState() => _HomePageState(); } class _HomePageState extends State<HomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Row(children: [ Image.asset( 'assets/logo.png', height: 30, ), Text('flutterflux.com') ]), ), body: Center( child: ElevatedButton( onPressed: () { ScaffoldMessenger.of(context).showSnackBar( SnackBar( content: CustomSnackBar(message: 'Custom SnackBar'), duration: Duration(seconds: 2), ), ); }, child: Text('show snackbar')), )); } } class CustomSnackBar extends StatefulWidget { final String message; CustomSnackBar({required this.message}); @override _CustomSnackBarState createState() => _CustomSnackBarState(); } class _CustomSnackBarState extends State<CustomSnackBar> { @override Widget build(BuildContext context) { return Container( height: 50, decoration: BoxDecoration( color: Colors.deepPurple, borderRadius: BorderRadius.circular(10), ), child: Row( mainAxisAlignment: MainAxisAlignment.spaceAround, children: [ SizedBox(width: 10), Text( widget.message, style: TextStyle(color: Colors.white, fontSize: 16), ), Icon(Icons.check, color: Colors.white), ], ), ); } }
Output

Snackbar without Scaffold
SnackBar in Flutter can be displayed even without a Scaffold.
import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'SnackBar in Flutter', home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Row(children: [ Image.asset( 'assets/logo.png', height: 30, ), Text('flutterflux.com') ]), ), body: Center( child: Builder( builder: (BuildContext context) { return ElevatedButton( onPressed: () { final snackBar = SnackBar( backgroundColor: Colors.deepPurple,//snackbar backgroundcolor content: Text('This is a SnackBar!'), ); ScaffoldMessenger.of(context).showSnackBar(snackBar); }, child: Text('Show SnackBar'), ); }, ), ), ); } }
A BuildContext
is provided by the Builder
widget, which is a descendant of the Scaffold widget, itself descended from Material. ScaffoldMessenger
can be contacted using this BuildContext
. It is possible to display the SnackBar by first calling of(context) to obtain the ScaffoldMessenger for the Scaffold, and then calling showSnackBar()
on the ScaffoldMessenger
.
Specifically, a Builder widget can be used to supply a BuildContext
if the latter is a descendant of a widget with a Material parent. One way to display a SnackBar is with a Builder widget, as example here.
Conclusion
Flutter SnackBar widget can show quick messages in reaction to user activities. It’s easy to apply and may be adjusted to match the app’s style. The SnackBar in Flutter can alert users to mistakes, warnings, and successful actions. It enhances the user experience and simplifies the software.